Syncfusion Essential PDF is a .NET PDF library that can be used to optimize or compress your PDF documents. Reducing the PDF file size can help you by optimizing bandwidth cost, network transmission, and digital storage. It is especially useful in areas like archiving, emailing, and using PDF documents in web-based applications. Essential PDF supports the following optimizations:
- Shrinking all images.
- Optimizing fonts.
- Removing metadata.
- Optimizing page content.
- Disabling incremental updates.
- Removing or flattening form fields.
- Removing or flattening annotations.
In this blog, we will look at each of these optimizations and how to implement them.

Experience a leap in PDF technology with Syncfusion's PDF Library, shaping the future of digital document processing.
Reducing PDF file size by shrinking all images
PDF files may contain many images. Removing the images from a PDF file is usually not an option since they are necessary for many PDFs. Downsampling the images will decrease the number of pixels and is possibly the most effective way to reduce PDF file size. The user can control the PDF file size with respect to the quality of the image.
//Create a new compression option object. PdfCompressionOptions options = new PdfCompressionOptions(); options.CompressImages = true; //Image quality is a percentage. options.ImageQuality = 10;
Reducing PDF file size by optimizing fonts
PdfCompressionOptions options = new PdfCompressionOptions(); options.OptimizeFont = true;

Boost your productivity with Syncfusion's PDF Library! Gain access to invaluable insights and tutorials in our extensive documentation.
Reducing PDF file size by removing metadata
Sometimes unnecessary metadata might be present in the PDF file. Remove the unnecessary metadata, to reduce the size of the PDF.
PdfCompressionOptions options = new PdfCompressionOptions(); options.RemoveMetadata = true;
Reducing PDF file size by optimizing page contents
Optimizing the page contents will remove unwanted commented lines, white spaces, convert end-of-line characters to spaces and compress all uncompressed contents.
PdfCompressionOptions options = new PdfCompressionOptions(); options.OptimizePageContents = true;
Reducing PDF file size by disabling incremental updates
Incremental update is an option available to update the PDF file incrementally without rewriting the entire file. Here, changes are appended to the end of the file, leaving its original contents intact. Disabling the incremental update will rewrite the entire file, which results in a smaller PDF.
//Load the existing PDF document. PdfLoadedDocument loadedDocument = new PdfLoadedDocument(@"input.pdf"); //Restructure the complete document. loadedDocument.FileStructure.IncrementalUpdate = false;

See a world of document processing possibilities in Syncfusion's PDF Library as we unveil its features in interactive demonstrations.
Reducing PDF file size by removing form fields
Removing or flattening form fields can help reduce your file size. When form fields and their values are not necessary, they can be removed. When they are necessary but require no further editing, they can be flattened. Both of these actions will result in a reduced file size.
public void RemoveFormFields(PdfLoadedDocument ldoc, bool flatten) { if (flatten) { ldoc.Form.Flatten = true; } else { int count = ldoc.Form.Fields.Count; for (int i = count - 1; i >= 0; i--) { ldoc.Form.Fields.RemoveAt(i); } } }
Reducing PDF file size by removing annotations
Removing or flattening annotations can help reduce your file size. Annotations and their contents can be removed when they are not needed. When they are necessary but do not require additional editing, they can be flattened. Both of these options will result in a reduced file size.
public void RemoveAnnotations(PdfLoadedDocument ldoc, bool flatten) { foreach(PdfPageBase page in ldoc.Pages) { if (flatten) { page.Annotations.Flatten = true; } else { int count = page.Annotations.Count; for (int i = count - 1;i >= 0; i--) { page.Annotations.RemoveAt(i); } } } }
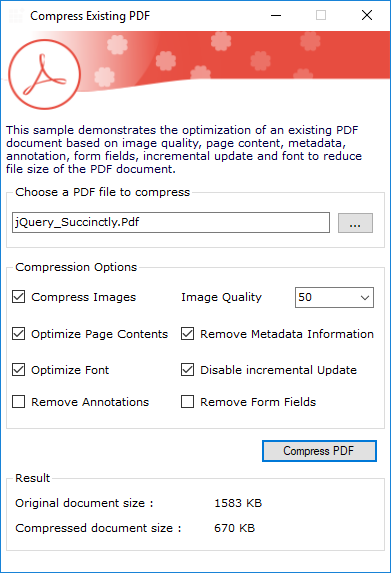
GitHub sample
For more details about this sample, refer to the complete compress PDF application demo on the GitHub repository.

Syncfusion’s high-performance PDF Library allows you to create PDF documents from scratch without Adobe dependencies.
Wrapping up
As you can see, Essential PDF provides a variety of optimizing mechanisms for compressing a PDF document. Use them effectively to generate compressed documents for increased efficiency and productivity in a document management system. Take a moment to peruse the documentation, where you’ll find other options and features, all with accompanying code examples.
If you are new to our PDF library, it is highly recommended that you follow our Getting Started guide.
If you’re already a Syncfusion user, you can download the product setup here else, you can download a free, 30-day trial here.
Have any questions or require clarifications about these features? Please let us know in the comments below, you can also contact us through our support forum or Direct-Trac. We are happy to assist you!
If you liked this blog post, we think you’ll also like the following related blog posts:
Comments (2)
Is it possible to reduce a pdf document 92, 032 KB to 1, 749 KB?
I need to integrate it into my application developed at home with winforms vb.net
Hi,
Yes, we can compress a PDF file from 92 MB to 1.749 MB but it is based on the input document structure, usually we will compress PDF inner objects which is already not in the best compression.
For example if a document has more number of images with low compression then we can compress the document further by shrinking all the images to best compression also we can use other options provided in this blog
• Optimizing fonts.
• Removing metadata.
• Optimizing page content.
• Disabling incremental updates.
• Removing or flattening form fields.
• Removing or flattening annotations.
Usually we cannot further compress the PDF document if it is already in the best compression state.
Regards,
Praveen
Comments are closed.